在这篇文章中,我们将深入探讨如何在C#中使用HttpClient.PostAsync
进行HTTP POST请求。我们将涵盖基础知识、一些高级用法以及实际应用示例。让我们开始吧!
什么是HttpClient?
HttpClient
是.NET库中的一个类,用于处理HTTP请求。它可以让你发送数据到服务器或从服务器获取数据。
使用HttpClient的优势
异步操作:确保你的应用在等待响应时不会冻结。
可重用:你可以使同一个实例进行多次请求。
灵活性:支持多种HTTP方法和可定制的头信息。
HttpClient.PostAsync的定义和用途
HttpClient.PostAsync
基本上是告诉你的程序使用HTTP POST方法异步地向指定的URL发送数据。想象一下,它就像是即时可靠地邮寄一封信。
何时使用PostAsync
当你需要向服务器发送数据以创建或更新资源时,使用PostAsync
。这就像提交表单或上传文件。
基本语法和用法
以下是一个简单的示例:
HttpClient client = new HttpClient();
User user = new User();
user.name = "张三";
user.city = "北京";
string jsonData = System.Text.Json.JsonSerializer.Serialize(user);
HttpContent content = new StringContent(jsonData, Encoding.UTF8, "application/json");
HttpResponseMessage response = await client.PostAsync("https://localhost:7144/api/User/Add", content);
Console.WriteLine(await response.Content.ReadAsStringAsync());
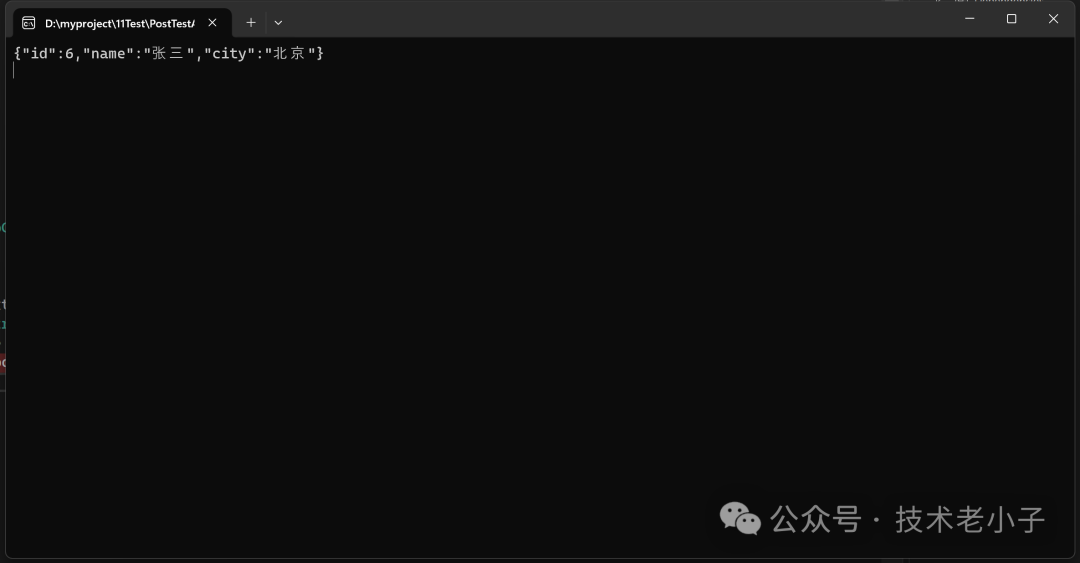
在这个代码片段中,jsonData
是你的JSON格式的数据。这就像是在发送包裹前进行包装。
如何执行基本的POST请求
发送简单数据
让我们动手实践一下,以下是如何发送简单的字符串数据:
var client = new HttpClient();
var content = new StringContent("This is some data", Encoding.UTF8, "text/plain");
HttpResponseMessage response = await client.PostAsync("https://localhost:7144/api/User/post", content);
Console.WriteLine(await response.Content.ReadAsStringAsync());
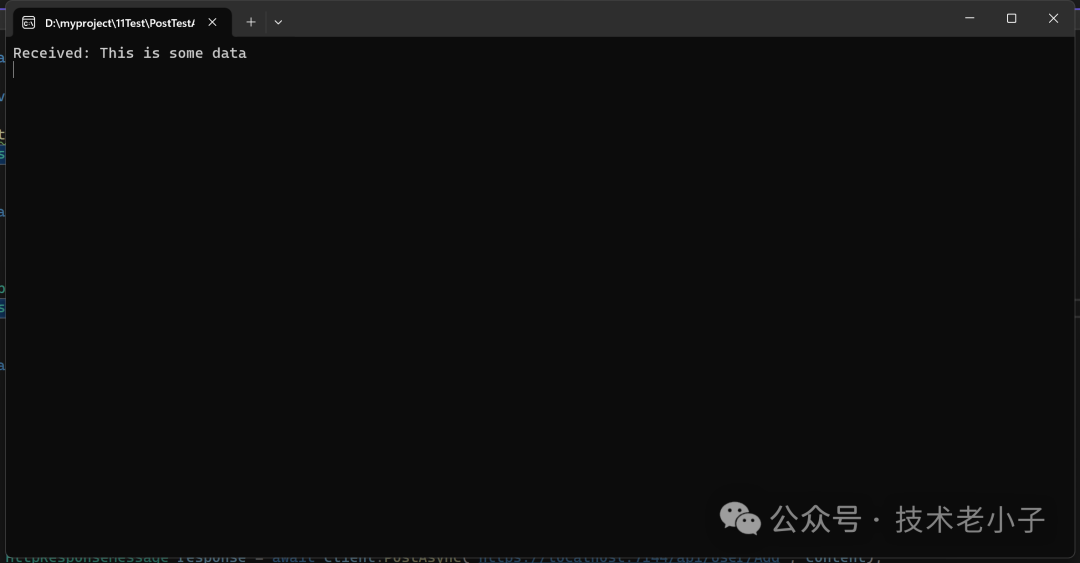
处理表单数据
有时,你需要发送表单数据,就像在网页上提交表单一样。以下是操作方法:
HttpClient client = new HttpClient();
var form = new MultipartFormDataContent();
form.Add(new StringContent("John"), "name");
form.Add(new StringContent("New York"), "city");
var response = await client.PostAsync("https://localhost:7144/api/User/Post", form);
Console.WriteLine(await response.Content.ReadAsStringAsync());
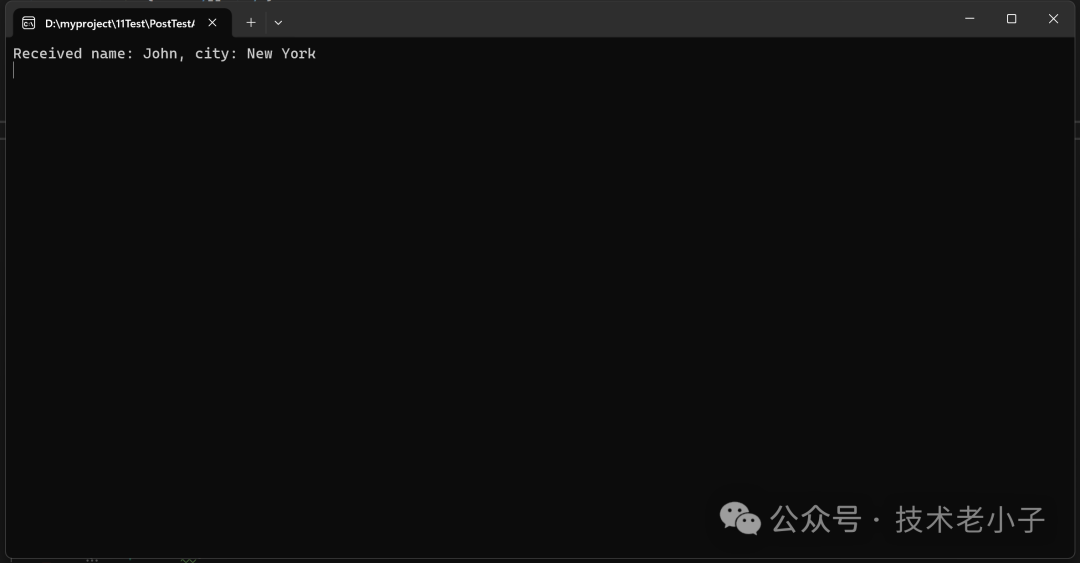
HttpClient.PostAsync的高级用法
添加请求头
请求头就像包裹上的标签,提供额外的元数据:
var client = new HttpClient();
client.DefaultRequestHeaders.Add("Authorization", "Bearer?YOUR_TOKEN_HERE");
使用身份验证
当你需要证明你是谁时,使用身份验证。以下是一个基本示例:
var client = new HttpClient();
var byteArray = Encoding.ASCII.GetBytes("username:password");
client.DefaultRequestHeaders.Authorization = new System.Net.Http.Headers.AuthenticationHeaderValue("Basic", Convert.ToBase64String(byteArray));
处理不同的内容类型
除了JSON,你可能需要处理XML或其他格式:
HttpContent content = new StringContent("<xml><name>John</name></xml>", Encoding.UTF8, "application/xml");
HttpResponseMessage response = await client.PostAsync("https://example.com/api", content);
错误处理
常见错误和异常
错误处理最佳实践
始终检查响应状态。以下是高效捕获错误的方法:
try
{
var response = await client.PostAsync(uri, content);
response.EnsureSuccessStatusCode();
}
catch (HttpRequestException e)
{
Console.WriteLine($"Request error: {e.Message}");
}
性能优化
高效的连接管理
重用HttpClient
实例以优化性能:
static readonly HttpClient client = new HttpClient();
优化高吞吐量
对于高容量请求,最小化阻塞:
var tasks = new List<Task<HttpResponseMessage>>();
foreach (var item in items)
{
tasks.Add(client.PostAsync(uri, new StringContent(item)));
}
await Task.WhenAll(tasks);
异步编程最佳实践
异步方法应正确等待以避免死锁。
实际应用示例
创建一个REST客户端
假设你想创建一个可重用的REST客户端。以下是骨架代码:
public class RestClient
{
private static readonly HttpClient client = new HttpClient();
public async Task<string> PostDataAsync(string uri, string jsonData)
{
var content = new StringContent(jsonData, Encoding.UTF8, "application/json");
var response = await client.PostAsync(uri, content);
response.EnsureSuccessStatusCode();
return await response.Content.ReadAsStringAsync();
}
}
RestClient client = new RestClient();
User user = new User();
user.name = "张三";
user.city = "北京";
string jsonData = System.Text.Json.JsonSerializer.Serialize(user);
string ret = await client.PostDataAsync("https://localhost:7144/api/User/Add",jsonData);
Console.WriteLine(ret);
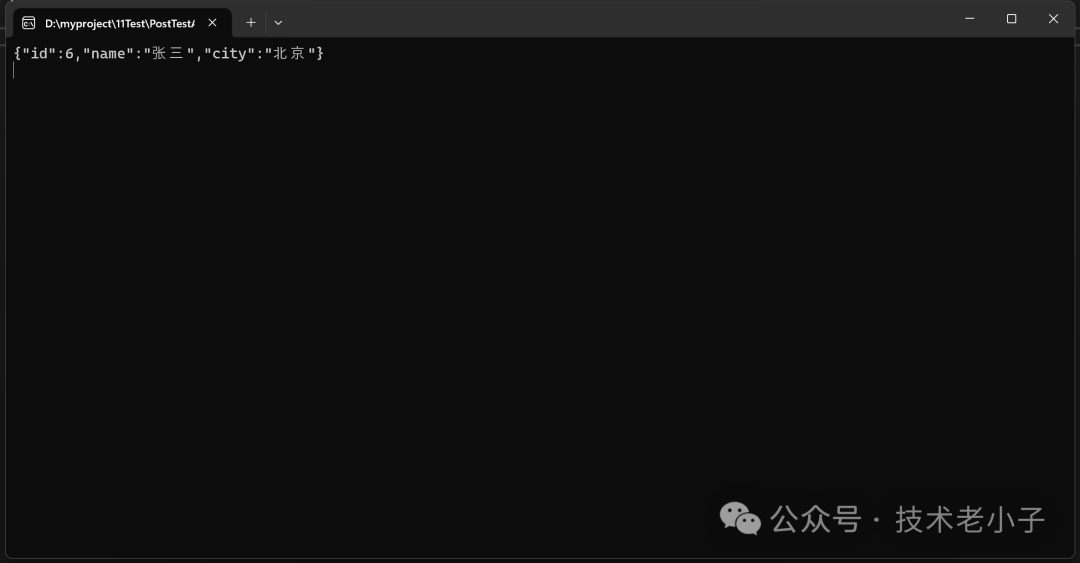
上传文件
需要上传文件?以下是操作方法:
var form = new MultipartFormDataContent();
var fileContent = new ByteArrayContent(File.ReadAllBytes("filePath"));
form.Add(fileContent, "file", "fileName.txt");
var response = await client.PostAsync("https://example.com/upload", form);
故障排除
调试常见问题
问题可能会让你困惑,但像Fiddler和Postman这样的工具可以拯救你。它们允许你检查HTTP请求和响应。
故障排除工具和技术
结论
HttpClient.PostAsync
是一个在C#中进行POST请求的强大工具。它简单、灵活且功能强大。从简单的数据提交到复杂的身份验证请求,HttpClient
都能胜任。探索这个多功能工具将为你的C#项目打开新的可能性,使你的网络交互更加顺畅和高效。
该文章在 2024/7/31 12:43:00 编辑过